2014-04-02 15:28:08 +00:00
|
|
|
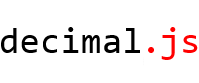
|
|
|
|
|
|
|
|
An arbitrary-precision Decimal type for JavaScript.
|
2016-02-04 23:52:52 +00:00
|
|
|
|
2020-09-28 21:28:15 +00:00
|
|
|
[](https://www.npmjs.com/package/decimal.js)
|
|
|
|
[](https://www.npmjs.com/package/decimal.js)
|
2014-11-11 10:44:42 +00:00
|
|
|
[](https://travis-ci.org/MikeMcl/decimal.js)
|
2017-01-02 16:20:11 +00:00
|
|
|
[](https://cdnjs.com/libraries/decimal.js)
|
2017-05-04 12:05:38 +00:00
|
|
|
|
2016-02-29 19:24:47 +00:00
|
|
|
<br>
|
|
|
|
|
2014-04-02 15:28:08 +00:00
|
|
|
## Features
|
|
|
|
|
2016-02-29 19:24:47 +00:00
|
|
|
- Integers and floats
|
2016-01-25 00:11:32 +00:00
|
|
|
- Simple but full-featured API
|
|
|
|
- Replicates many of the methods of JavaScript's `Number.prototype` and `Math` objects
|
|
|
|
- Also handles hexadecimal, binary and octal values
|
2014-04-02 15:28:08 +00:00
|
|
|
- Faster, smaller, and perhaps easier to use than JavaScript versions of Java's BigDecimal
|
|
|
|
- No dependencies
|
2014-04-10 18:30:38 +00:00
|
|
|
- Wide platform compatibility: uses JavaScript 1.5 (ECMAScript 3) features only
|
2021-02-16 12:37:43 +00:00
|
|
|
- Comprehensive [documentation](https://mikemcl.github.io/decimal.js/) and test set
|
2017-12-03 18:09:41 +00:00
|
|
|
- Includes a TypeScript declaration file: *decimal.d.ts*
|
2014-04-02 15:28:08 +00:00
|
|
|
|
|
|
|

|
|
|
|
|
|
|
|
The library is similar to [bignumber.js](https://github.com/MikeMcl/bignumber.js/), but here
|
2016-11-09 17:08:38 +00:00
|
|
|
precision is specified in terms of significant digits rather than decimal places, and all
|
2014-04-02 15:28:08 +00:00
|
|
|
calculations are rounded to the precision (similar to Python's decimal module) rather than just
|
|
|
|
those involving division.
|
|
|
|
|
2016-02-29 19:24:47 +00:00
|
|
|
This library also adds the trigonometric functions, among others, and supports non-integer powers,
|
|
|
|
which makes it a significantly larger library than *bignumber.js* and the even smaller
|
|
|
|
[big.js](https://github.com/MikeMcl/big.js/).
|
2014-04-02 15:28:08 +00:00
|
|
|
|
2017-12-10 18:32:38 +00:00
|
|
|
For a lighter version of this library without the trigonometric functions see [decimal.js-light](https://github.com/MikeMcl/decimal.js-light/).
|
2016-11-09 17:08:38 +00:00
|
|
|
|
2014-04-02 15:28:08 +00:00
|
|
|
## Load
|
|
|
|
|
2020-09-28 21:28:15 +00:00
|
|
|
The library is the single JavaScript file *decimal.js* or ES module *decimal.mjs*.
|
2014-04-02 15:28:08 +00:00
|
|
|
|
2017-12-10 18:32:38 +00:00
|
|
|
Browser:
|
2016-02-29 19:24:47 +00:00
|
|
|
|
2016-02-23 10:07:52 +00:00
|
|
|
```html
|
|
|
|
<script src='path/to/decimal.js'></script>
|
|
|
|
```
|
2016-02-29 19:24:47 +00:00
|
|
|
|
2020-09-28 21:28:15 +00:00
|
|
|
```html
|
|
|
|
<script type="module">
|
|
|
|
import Decimal from './path/to/decimal.mjs';
|
|
|
|
...
|
|
|
|
</script>
|
|
|
|
```
|
|
|
|
|
2021-02-16 12:37:43 +00:00
|
|
|
[Node.js](https://nodejs.org):
|
2017-12-10 18:32:38 +00:00
|
|
|
|
|
|
|
```bash
|
2020-09-28 21:28:15 +00:00
|
|
|
$ npm install decimal.js
|
2017-12-10 18:32:38 +00:00
|
|
|
```
|
2016-02-29 19:24:47 +00:00
|
|
|
|
|
|
|
```js
|
2016-03-02 19:50:36 +00:00
|
|
|
var Decimal = require('decimal.js');
|
2016-02-23 10:07:52 +00:00
|
|
|
```
|
2016-02-29 19:24:47 +00:00
|
|
|
|
2020-09-28 21:28:15 +00:00
|
|
|
ES module:
|
2016-02-29 19:24:47 +00:00
|
|
|
|
2017-12-10 18:32:38 +00:00
|
|
|
```js
|
|
|
|
//import Decimal from 'decimal.js';
|
|
|
|
import {Decimal} from 'decimal.js';
|
2016-02-29 19:24:47 +00:00
|
|
|
```
|
|
|
|
|
2021-02-16 12:37:43 +00:00
|
|
|
AMD loader libraries such as [requireJS](https://requirejs.org/):
|
2016-02-29 19:24:47 +00:00
|
|
|
|
|
|
|
```js
|
2016-02-23 10:07:52 +00:00
|
|
|
require(['decimal'], function(Decimal) {
|
|
|
|
// Use Decimal here in local scope. No global Decimal.
|
|
|
|
});
|
|
|
|
```
|
2016-02-29 19:24:47 +00:00
|
|
|
|
2014-04-02 15:28:08 +00:00
|
|
|
## Use
|
|
|
|
|
|
|
|
*In all examples below, `var`, semicolons and `toString` calls are not shown.
|
|
|
|
If a commented-out value is in quotes it means `toString` has been called on the preceding expression.*
|
|
|
|
|
2016-01-25 00:11:32 +00:00
|
|
|
The library exports a single function object, `Decimal`, the constructor of Decimal instances.
|
2014-04-02 15:28:08 +00:00
|
|
|
|
2016-01-25 00:11:32 +00:00
|
|
|
It accepts a value of type number, string or Decimal.
|
2016-02-29 19:24:47 +00:00
|
|
|
|
|
|
|
```js
|
2016-02-23 10:07:52 +00:00
|
|
|
x = new Decimal(123.4567)
|
|
|
|
y = new Decimal('123456.7e-3')
|
|
|
|
z = new Decimal(x)
|
|
|
|
x.equals(y) && y.equals(z) && x.equals(z) // true
|
|
|
|
```
|
2016-02-29 19:24:47 +00:00
|
|
|
|
2016-01-25 00:11:32 +00:00
|
|
|
A value can also be in binary, hexadecimal or octal if the appropriate prefix is included.
|
2016-02-29 19:24:47 +00:00
|
|
|
|
|
|
|
```js
|
2016-02-23 10:07:52 +00:00
|
|
|
x = new Decimal('0xff.f') // '255.9375'
|
|
|
|
y = new Decimal('0b10101100') // '172'
|
|
|
|
z = x.plus(y) // '427.9375'
|
2016-02-29 19:24:47 +00:00
|
|
|
|
2016-02-23 10:07:52 +00:00
|
|
|
z.toBinary() // '0b110101011.1111'
|
|
|
|
z.toBinary(13) // '0b1.101010111111p+8'
|
|
|
|
```
|
2016-02-29 19:24:47 +00:00
|
|
|
|
2017-12-03 18:09:41 +00:00
|
|
|
Using binary exponential notation to create a Decimal with the value of `Number.MAX_VALUE`:
|
|
|
|
|
|
|
|
```js
|
|
|
|
x = new Decimal('0b1.1111111111111111111111111111111111111111111111111111p+1023')
|
|
|
|
```
|
|
|
|
|
2014-04-02 15:28:08 +00:00
|
|
|
A Decimal is immutable in the sense that it is not changed by its methods.
|
2016-02-29 19:24:47 +00:00
|
|
|
|
|
|
|
```js
|
2016-02-23 10:07:52 +00:00
|
|
|
0.3 - 0.1 // 0.19999999999999998
|
|
|
|
x = new Decimal(0.3)
|
|
|
|
x.minus(0.1) // '0.2'
|
|
|
|
x // '0.3'
|
|
|
|
```
|
2016-02-29 19:24:47 +00:00
|
|
|
|
2014-04-02 15:28:08 +00:00
|
|
|
The methods that return a Decimal can be chained.
|
2016-02-29 19:24:47 +00:00
|
|
|
|
|
|
|
```js
|
2016-02-23 10:07:52 +00:00
|
|
|
x.dividedBy(y).plus(z).times(9).floor()
|
|
|
|
x.times('1.23456780123456789e+9').plus(9876.5432321).dividedBy('4444562598.111772').ceil()
|
|
|
|
```
|
2016-02-29 19:24:47 +00:00
|
|
|
|
2014-04-02 15:28:08 +00:00
|
|
|
Many method names have a shorter alias.
|
2016-02-29 19:24:47 +00:00
|
|
|
|
|
|
|
```js
|
2016-02-23 10:07:52 +00:00
|
|
|
x.squareRoot().dividedBy(y).toPower(3).equals(x.sqrt().div(y).pow(3)) // true
|
|
|
|
x.cmp(y.mod(z).neg()) == 1 && x.comparedTo(y.modulo(z).negated()) == 1 // true
|
|
|
|
```
|
2016-02-29 19:24:47 +00:00
|
|
|
|
2016-01-25 00:11:32 +00:00
|
|
|
Like JavaScript's Number type, there are `toExponential`, `toFixed` and `toPrecision` methods,
|
2016-02-29 19:24:47 +00:00
|
|
|
|
|
|
|
```js
|
2016-02-23 10:07:52 +00:00
|
|
|
x = new Decimal(255.5)
|
|
|
|
x.toExponential(5) // '2.55500e+2'
|
|
|
|
x.toFixed(5) // '255.50000'
|
|
|
|
x.toPrecision(5) // '255.50'
|
2016-02-29 19:24:47 +00:00
|
|
|
```
|
|
|
|
|
|
|
|
and almost all of the methods of JavaScript's Math object are also replicated.
|
|
|
|
|
|
|
|
```js
|
2016-02-23 10:07:52 +00:00
|
|
|
Decimal.sqrt('6.98372465832e+9823') // '8.3568682281821340204e+4911'
|
|
|
|
Decimal.pow(2, 0.0979843) // '1.0702770511687781839'
|
|
|
|
```
|
2016-02-29 19:24:47 +00:00
|
|
|
|
2016-01-25 00:11:32 +00:00
|
|
|
There are `isNaN` and `isFinite` methods, as `NaN` and `Infinity` are valid `Decimal` values,
|
2016-02-29 19:24:47 +00:00
|
|
|
|
|
|
|
```js
|
2016-02-23 10:07:52 +00:00
|
|
|
x = new Decimal(NaN) // 'NaN'
|
|
|
|
y = new Decimal(Infinity) // 'Infinity'
|
|
|
|
x.isNaN() && !y.isNaN() && !x.isFinite() && !y.isFinite() // true
|
2016-02-29 19:24:47 +00:00
|
|
|
```
|
|
|
|
|
2016-01-25 00:11:32 +00:00
|
|
|
and a `toFraction` method with an optional *maximum denominator* argument
|
2016-02-29 19:24:47 +00:00
|
|
|
|
|
|
|
```js
|
2016-02-23 10:07:52 +00:00
|
|
|
z = new Decimal(355)
|
|
|
|
pi = z.dividedBy(113) // '3.1415929204'
|
|
|
|
pi.toFraction() // [ '7853982301', '2500000000' ]
|
|
|
|
pi.toFraction(1000) // [ '355', '113' ]
|
|
|
|
```
|
2016-02-29 19:24:47 +00:00
|
|
|
|
2014-11-10 16:00:14 +00:00
|
|
|
All calculations are rounded according to the number of significant digits and rounding mode
|
2016-02-29 19:24:47 +00:00
|
|
|
specified by the `precision` and `rounding` properties of the Decimal constructor.
|
|
|
|
|
2018-03-10 22:00:51 +00:00
|
|
|
For advanced usage, multiple Decimal constructors can be created, each with their own independent configuration which
|
2016-02-29 19:24:47 +00:00
|
|
|
applies to all Decimal numbers created from it.
|
2014-04-10 18:30:38 +00:00
|
|
|
|
2016-02-29 19:24:47 +00:00
|
|
|
```js
|
2016-02-23 10:07:52 +00:00
|
|
|
// Set the precision and rounding of the default Decimal constructor
|
2016-11-09 17:08:38 +00:00
|
|
|
Decimal.set({ precision: 5, rounding: 4 })
|
2014-04-02 15:28:08 +00:00
|
|
|
|
2016-02-23 10:07:52 +00:00
|
|
|
// Create another Decimal constructor, optionally passing in a configuration object
|
2017-12-10 18:32:38 +00:00
|
|
|
Decimal9 = Decimal.clone({ precision: 9, rounding: 1 })
|
2014-04-02 15:28:08 +00:00
|
|
|
|
2016-02-23 10:07:52 +00:00
|
|
|
x = new Decimal(5)
|
2017-12-10 18:32:38 +00:00
|
|
|
y = new Decimal9(5)
|
2014-04-02 15:28:08 +00:00
|
|
|
|
2016-02-23 10:07:52 +00:00
|
|
|
x.div(3) // '1.6667'
|
2017-12-10 18:32:38 +00:00
|
|
|
y.div(3) // '1.66666666'
|
2016-02-23 10:07:52 +00:00
|
|
|
```
|
2016-02-29 19:24:47 +00:00
|
|
|
|
2016-01-25 00:11:32 +00:00
|
|
|
The value of a Decimal is stored in a floating point format in terms of its digits, exponent and sign.
|
2016-02-29 19:24:47 +00:00
|
|
|
|
|
|
|
```js
|
2016-02-23 10:07:52 +00:00
|
|
|
x = new Decimal(-12345.67);
|
2016-02-29 19:24:47 +00:00
|
|
|
x.d // [ 12345, 6700000 ] digits (base 10000000)
|
2016-02-23 10:07:52 +00:00
|
|
|
x.e // 4 exponent (base 10)
|
|
|
|
x.s // -1 sign
|
|
|
|
```
|
2016-02-29 19:24:47 +00:00
|
|
|
|
2014-04-02 15:28:08 +00:00
|
|
|
For further information see the [API](http://mikemcl.github.io/decimal.js/) reference in the *doc* directory.
|
|
|
|
|
|
|
|
## Test
|
|
|
|
|
2016-01-25 00:11:32 +00:00
|
|
|
The library can be tested using Node.js or a browser.
|
2014-04-02 15:28:08 +00:00
|
|
|
|
2016-02-29 19:24:47 +00:00
|
|
|
The *test* directory contains the file *test.js* which runs all the tests when executed by Node,
|
|
|
|
and the file *test.html* which runs all the tests when opened in a browser.
|
2014-04-02 15:28:08 +00:00
|
|
|
|
2016-01-25 00:11:32 +00:00
|
|
|
To run all the tests, from a command-line at the root directory using npm
|
2016-02-29 19:24:47 +00:00
|
|
|
|
2016-02-23 10:07:52 +00:00
|
|
|
```bash
|
|
|
|
$ npm test
|
|
|
|
```
|
2016-02-29 19:24:47 +00:00
|
|
|
|
2016-01-25 00:11:32 +00:00
|
|
|
or at the *test* directory using Node
|
2016-02-29 19:24:47 +00:00
|
|
|
|
|
|
|
```bash
|
2016-02-23 10:07:52 +00:00
|
|
|
$ node test
|
2016-02-29 19:24:47 +00:00
|
|
|
```
|
|
|
|
|
2016-01-25 00:11:32 +00:00
|
|
|
Each separate test module can also be executed individually, for example, at the *test/modules* directory
|
2016-02-29 19:24:47 +00:00
|
|
|
|
2016-02-23 10:07:52 +00:00
|
|
|
```bash
|
|
|
|
$ node toFraction
|
|
|
|
```
|
2014-04-02 15:28:08 +00:00
|
|
|
|
|
|
|
## Build
|
|
|
|
|
|
|
|
For Node, if [uglify-js](https://github.com/mishoo/UglifyJS2) is installed
|
2016-02-29 19:24:47 +00:00
|
|
|
|
2016-02-23 10:07:52 +00:00
|
|
|
```bash
|
|
|
|
npm install uglify-js -g
|
|
|
|
```
|
2016-02-29 19:24:47 +00:00
|
|
|
|
2014-04-02 15:28:08 +00:00
|
|
|
then
|
2016-02-29 19:24:47 +00:00
|
|
|
|
2016-02-23 10:07:52 +00:00
|
|
|
```bash
|
|
|
|
npm run build
|
|
|
|
```
|
2016-02-29 19:24:47 +00:00
|
|
|
|
2016-01-25 00:11:32 +00:00
|
|
|
will create *decimal.min.js*, and a source map will also be added to the *doc* directory.
|
2014-04-02 15:28:08 +00:00
|
|
|
|
|
|
|
## Licence
|
|
|
|
|
2016-11-09 17:08:38 +00:00
|
|
|
MIT.
|
2014-04-02 15:28:08 +00:00
|
|
|
|
2016-01-25 00:11:32 +00:00
|
|
|
See *LICENCE.md*
|